Can field definitions affect performance?
Friday, September 29, 2006
I’ve been always curious if the field definitions (DATA) could affect the performance, especially in the subroutines. In some languages the field definition actually compiles into 2 operations: definition itself and field initialization. It is obvious that in this case the less field definition the better, but I wasn’t completely sure about ABAP.
Today I had to make a change in the program where about 20 fields were defined inside a routine, which was called in a loop, so I finally decided to find out whether it would make any difference if I moved the field definitions outside of the loop.
For a clean test, I wrote a simple program:
DO 100 TIMES.
PERFORM routine.
ENDDO.
FORM routine.
DATA: w_matnr TYPE matnr,
w_posnr TYPE posnr.
WRITE: w_matnr.
ENDFORM.
Then I changed it like this:
DATA: w_matnr TYPE matnr,
w_posnr TYPE posnr.
DO 100 TIMES.
PERFORM routine.
ENDDO.
FORM routine.
WRITE: w_matnr.
ENDFORM.
I ran Runtime Analysis on both several times to get more accurate results (that really drives me crazy that you get different results almost every time) and here is the average “before” picture
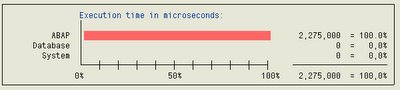
and “after” picture.

Well, it’s not huge but now I know that it does make a difference. What’s interesting though – the runtime did not increase much when I changed the DO cycle to 1000 times. Hmm... If anyone can explain this, please let me know.
posted by Your Friendly ABAPer @ 22:14,
Direct link to this post
Sending emails from SAP
Thursday, September 28, 2006
Today I was trying to set up email notification in one of my programs. There was a requirement to send out an email to a designated user if any errors occurred in the program. Fortunately, I already knew which function module to use (SO_NEW_DOCUMENT_SEND_API1), so I thought it would be a piece of cake.
In the documentation for that function module there is even a piece of code that illustrates how this FM should be used. So I just copy-pasted that code in my temporary program, added my email address and ran it. It did successfully send a message to my SAP Inbox but the email was not sent. After some checking around it turned out that we simply can not send emails from the test client (apparently the Basis guys were too lazy to set it up there). OK, no big deal – I ran my program in the right environment. And – hurrah! – got a message ‘...sent successfully’.
After 2 cups of coffee I suddenly realized that I should have gotten an email by now. But there was none... Hmm... More googling and more asking around brought the following results: if there was an email waiting to be sent I would have seen it in the transaction SCOT. Also the emails are actually sent out from SAP by the program RSCONN01, which is usually scheduled as a background job to run every N minutes. My program ran successfully and the job was running OK but SCOT was showing only bunch of zeroes. What the heck is going on?! So I went to check my program again, maybe I was missing something. Indeed I was! Turns out that the bast... , I mean nice person who wrote a code example in the FM documentation forgot about a little tiny detail – it needs COMMIT WORK, otherwise nothing will happen! It will give you all the “success” messages and sy-subrc = 0 but good luck waiting for that email to arrive.
In the process (I spent like an hour on this!) I’ve also managed to strip down the code to the bare minimum: email address, subject line and email body. Here is what it boiled down to:
DATA: objcont LIKE solisti1 OCCURS 0 WITH HEADER LINE.
DATA: reclist LIKE somlreci1 OCCURS 0 WITH HEADER LINE.
DATA: doc_chng LIKE sodocchgi1.
* Email subject line
doc_chng-obj_descr = 'Email subject'.
* Email body
objcont = 'This is a line to be sent in the email body'.
APPEND objcont.
* Receiver list
reclist-receiver = 'your email address here'.
reclist-rec_type = 'U'.
APPEND reclist.
* Send the document
CALL FUNCTION 'SO_NEW_DOCUMENT_SEND_API1'
EXPORTING
document_data = doc_chng
commit_work = 'X'
TABLES
object_content = objcont
receivers = reclist
EXCEPTIONS
too_many_receivers = 1
document_not_sent = 2
operation_no_authorization = 4
OTHERS = 99.
IF sy-subrc <> 0.
MESSAGE 'Email could not be sent' TYPE 'I'.
ELSE.
MESSAGE 'Email was sent successfully' TYPE 'I'.
ENDIF.
A few pointers:
- Email address is not case-sensitive.
- Since I have only one recipient, I check only for sy-subrc after the function module. If you have a list of recipients, use the code from the FM documentation, which checks the status of each recipient.
- Objcont here is an internal table. In my program I populate a table i_errors with the error messages and then copy the content to objcont.
You can also send attachments using this function module. As far as I understand, the attachment should be written into a file and the file name should be submitted to the FM.
I’m pretty sure you could get an email address from a user profile (user profile display is in transaction SU3) but we don’t store emails there, so we chose to use our own Z table instead.
posted by Your Friendly ABAPer @ 21:13,
Direct link to this post